Blackjack Codingbat Solution
Ternary Operator - BlackJack. A question from codingBat. Given 2 int values greater than 0, return whichever value is nearest to 21 without going over. Return 0 if they both go over. Blackjack(19, 21) → 21 blackjack(21, 19) → 21 blackjack(19, 22) → 19 Solution.
- CodingBat Java Logic-2 blackjack Given 2 int values greater than 0, return whichever value is nearest to 21 without going over. Return 0 if they both go over. Blackjack (19, 21) → 21.
- Solutions to CodingBat problems. Contribute to mirandaio/codingbat development by creating an account on GitHub.
The following tutorial is only some kind of “thought provoking” one: shows you some logic and class structure using enums and dynamically created images with Winform application.
Maybe I skipped some of the official rules. If so, I’m sorry I’m not a big gambler. This tutorial is not for teaching you Blackjack, but showing you some C# practices.
Here are some of the rules I have implemented:
- we have a shuffled deck of 52 cards
- the player starting hand has 2 cards
- the computer hand has also 2 cards: one of them is visible the other one is not
- the Jack, Queen and King has the value of 10
- the Ace has the value of 11 or 1 if 11 would be too much in hand
- the player starts to draw (official phrase is HIT)
- the main goal is to stop at 21 (or close to 21)
- the player can skip the drawing phase (STAY) if the total score is 16 at least
- if player stays, the computer turn comes
- first we can see the computer’s second (hidden) card
- the computer starts to draw using the same rules as ours mentioned before
To show you how to use System.Drawing.Graphics class, I create the images of the cards by cutting 1 big image into pieces. Click on the following image to download it:


I have 3 main classes: Card, Deck and Hand. Of course the form has its own Form1 class.

I also have 2 enums: CardValue and CardSuit. Both enum indexing starts from 1. The CardValue contains the type of the cards (Ace, King, 9, 7, 2, etc.). The CardSuit contains the colors (hearts, spades, etc.).
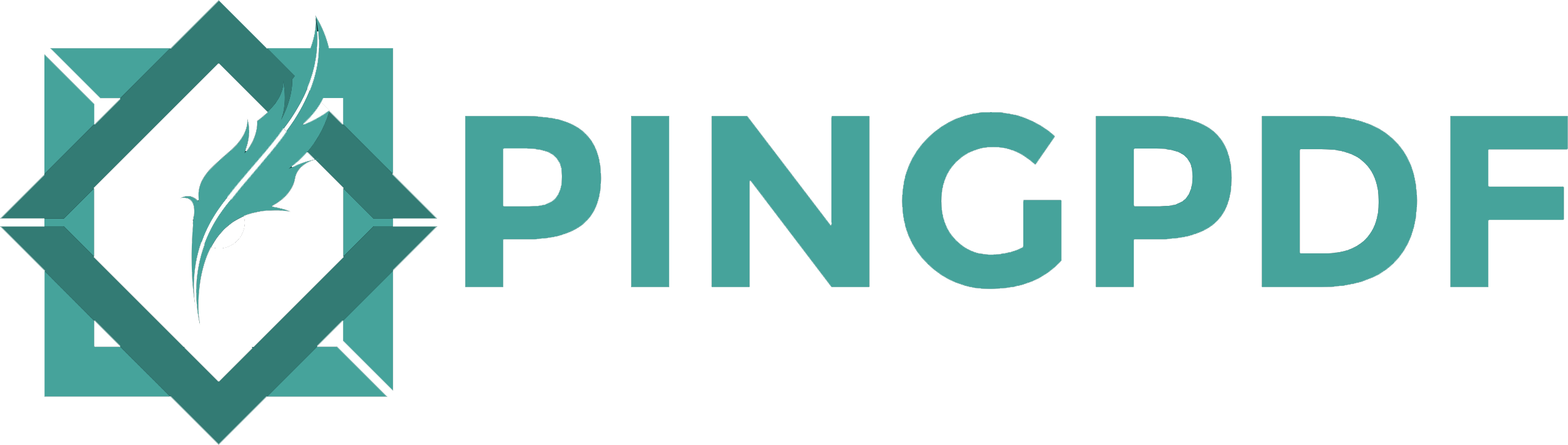
Create a new class: Card.cs
Under the class place the 2 enums:
The Card class will have 3 properties, 1 constructor, 1 new method and 1 overridden method. What properties does a card have? Of course its value, its color and its picture. So create the private properties with their public encapsulated fields. The Image property won’t have setter, the value and color setter will have the image loader method:
Blackjack Codingbat Solutions
With the constructor let’s create a dummy card with no color and value:
Codingbat Blackjack Solution
The attached image has the following properties: it’s 392 pixel high and 950 wide. So 1 card is about 97 high and 73 wide. The method has to slice the image depending on the following color from the deck (one color in each row on the image) and depending on the value (the image has the values in ascending order).
And the overridden ToString method, just for fun (not used later in the code):
In the next chapter I’ll show you how to create the class for the Hand and the Deck.